Computer Science 1
Clay Shields, Department of Computer Science, Georgetown University
Project 1 - Clyde's Carryout Cupcakes
Part 1: Project Design due September 27th, before class by
email or in class
Part 2: Due October 4th before class by electronic submission.
Congratulations! You have been hired by Clyde's Restaurant Group to provide computer support.
The first project you are going to work on is Clyde's Carryout Cupcakes (CCC). Clyde's is attempting to capitalize on the upscale cupcake craze by turning the 1789 pastry kitchen (located between F. Scotts and Wisey's seen under construction in the accompanying picture) into a carryout cupcake store. They need you to write some software that will help them ring up the cupcake purchases. Fortunately, the menu is pretty simple.
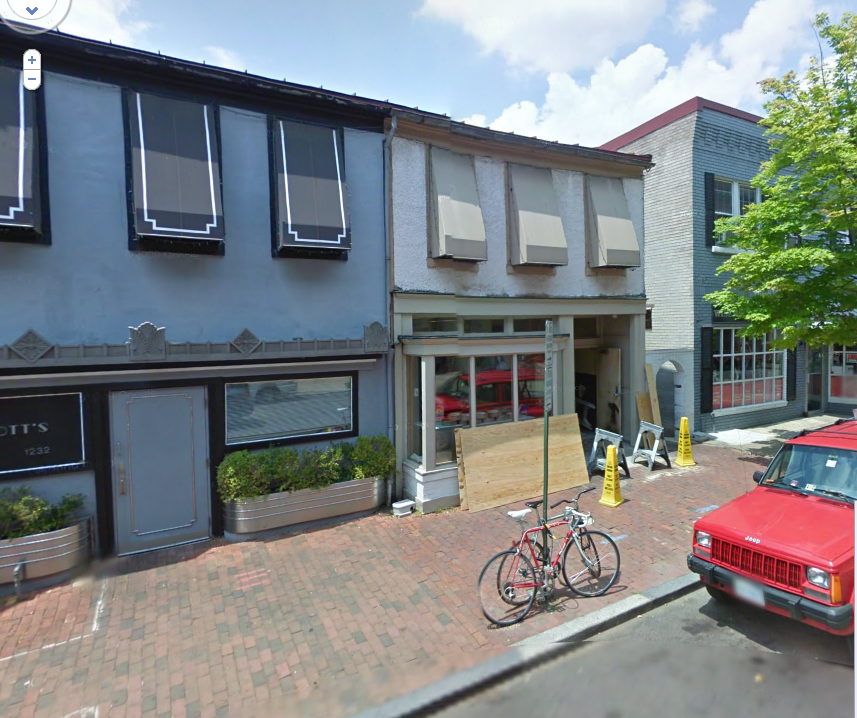
Clyde's Carryout Cupcakes offers only cupcakes. Each cupcake is $2.75. A dozen discounted to $30. Customers have the option of adding sprinkles to cupcakes at $0.35 apiece.
Your task is to write a program that will calculate the cost of the cupcakes that someone buys. It should correctly determine how many dozens there are, how many left that are not part of a dozen, and how many have sprinkes, then compute the total cost. It should then ask if the customer is in time for the Early Bird Gets the Cupcake special, which provides customers who arrive before 11:45 with an 15% discount. As one of the major customers is expected to be Georgetown University, it should ask if the cupcake purchase is for an official GU function; if so, then it should not charge the usual 10% sales tax. CCC can only make about 1,200 cupcakes a day, so don't let people order more than that.
For output, your program should only show the relevant lines. For example, if there is an order with one dozen cupcakes, the individual cupcake cost should be hidden. If there is one cupcake, the dozens should be hidden. If there are no sprinkles, that should be hidden. The exception is taxes - always show the tax amount.
Here is some sample output from a program that implements the
algorithm required for this project:
Welcome to Clyde's Carryout Cupcakes!
Please enter the following information:
Enter the number of cupcakes purchased: 197
How many cupcakes had sprinkles: 12
Was this order placed before 11:45 (y/n)? y
Is this order tax exempt, such as a GU official purchase (y/n)? n
Clyde's Carryout Cupcakes - Thanks for your order!
Dozens ordered: 16 @ $480.00
Individual cupcakes: 5 @ $13.75
Cupcake sprinkles: 12 @ $4.20
Subtotal: $497.95
Early bird discount: -$74.69
Subtotal with discount: $423.26
Tax: $42.33
Please pay: $465.58
Please note the neat alignment of the output.
You will be able to experiment with the program above to test your
own against it. To do so, run the following commands on seva.
cp ~clay/ccc.exe .
./ccc.exe
This will copy the working program to your directory where you can run it.
Part 1 - Design Document
For the first part you are to submit a design document showing the algorithm you plan to implement. DO NOT GIVE ME A FLOWCHART. Instead, write it out neatly using a language which is similar to that from Homework 1 and has the following terms:- input
- output
- calculate
- if condition, then statement
- if condition, then statement; otherwise, statement
- start
- stop
-
begin
statement
...
statement
end
Part 2 - Program Source Code
Important: Your output and input should be very similar to that shown in the example program. Please ask for the input in exactly the same order shown and only request the same items shown - do not ask for any other input. This will assist in grading your program.I expect your program to have the following features, similar to the one that you can run to compare your program to:
- All input must be checked to make sure it is reasonable. For now, if you detect unreasonable input, the program can just print an error message and halt.
- You should declare values that are fixed as const and use the declared constants in your calculations.
- Your output should be neat and aligned similarly to that of the example above and of the example program.
Include the following header in your source code.
//
// Project 1
// Name: <your name>
// E-mail: <your e-mail address>
// COSC 071
//
// In accordance with the class policies and Georgetown's Honor Code,
// I certify that I have neither given nor received any assistance
// on this project with the exceptions of the lecture notes and those
// items noted below.
//
// Description: <Describe your program>
//
You will submit your
To submit your program, make sure there is a copy of the source code on your account on seva. You may name your program what you like - let's assume that it is called cupcake.cc. To submit your program electronically, use the submit program like we did in Homework 2, but with the command:
java -jar submit.jar -a p1 -f cupcake.cc
I will not be enabling the electronic submission until after the design documents are in, so don't try to submit too early.